Tools Dependency Injection
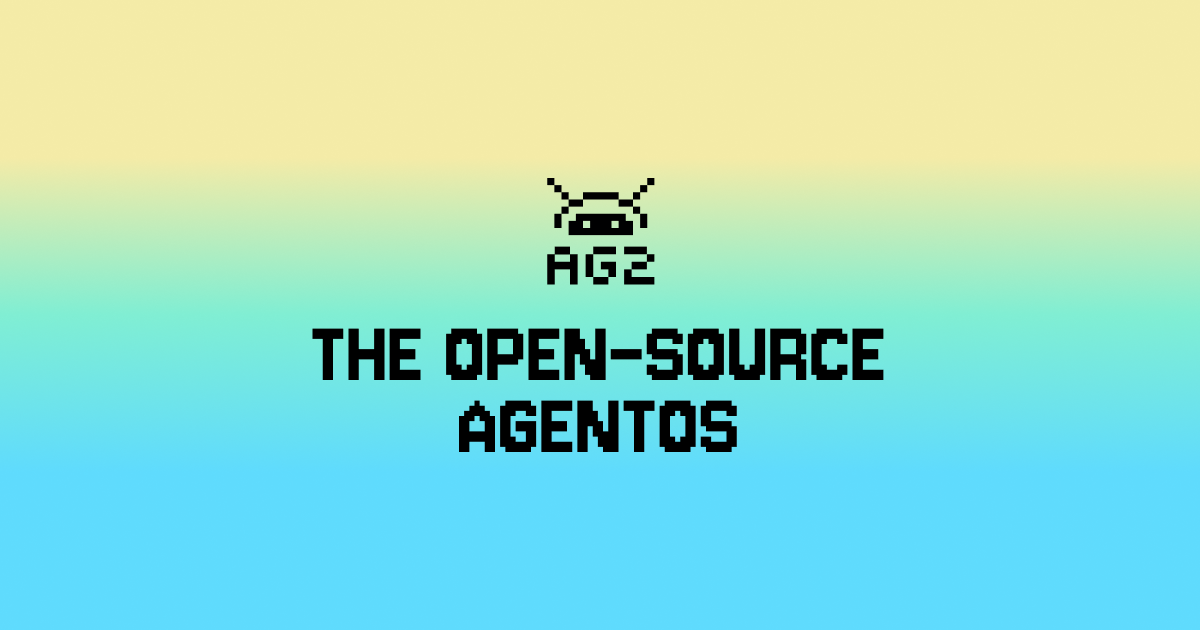
Dependency Injection is a secure way to connect external functions to agents without exposing sensitive data such as passwords, tokens, or personal information. This approach ensures that sensitive information remains protected while still allowing agents to perform their tasks effectively, even when working with large language models (LLMs).
In this guide, we’ll explore how to build secure workflows that handle sensitive data safely.
As an example, we’ll create an agent that retrieves user’s account balance. The best part is that sensitive data like username and password are never shared with the LLM. Instead, it’s securely injected directly into the function at runtime, keeping it safe while maintaining seamless functionality.
Why Dependency Injection Is Essential
Here’s why dependency injection is a game-changer for secure LLM workflows:
- Enhanced Security: Your sensitive data is never directly exposed to the LLM.
- Simplified Development: Secure data can be seamlessly accessed by functions without requiring complex configurations.
- Unmatched Flexibility: It supports safe integration of diverse workflows, allowing you to scale and adapt with ease.
In this guide, we’ll explore how to set up dependency injection and build secure workflows. Let’s dive in!
Note: This blog builds upon the concepts covered in the following notebook.
Installation
To install AG2
, simply run the following command:
Imports
The functionality demonstrated in this guide is located in the autogen.tools.dependency_injection
module. This module provides key components for dependency injection:
BaseContext
: abstract base class used to define and encapsulate data contexts, such as user account information, which can then be injected into functions or agents securely.Depends
: a function used to declare and inject dependencies, either from a context (likeBaseContext
) or a function, ensuring sensitive data is provided securely without direct exposure.
Define a BaseContext Class
We start by defining a BaseContext
class for accounts. This will act as the base structure for dependency injection. By using this approach, sensitive information like usernames and passwords is never exposed to the LLM.
Helper Functions
To ensure that the provided account is valid and retrieve its balance, we create two helper functions.
Injecting BaseContext Parameter
Dependency injection simplifies passing data to a function. Here, we’ll inject an Account
instance into a function automatically.
Agent Configuration
Configure the agents for the interaction.
config_list
defines the LLM configurations, including the model and API key.UserProxyAgent
simulates user inputs without requiring actual human interaction (set toNEVER
).AssistantAgent
represents the AI agent, configured with the LLM settings.
Register the Function with Dependency Injection
We register a function where the account information for bob
is injected as a dependency.
Note: You can also use account: Account = Depends(bob_account)
as an alternative syntax.
Initiate the Chat
Finally, we initiate a chat to retrieve the balance.
Injecting Parameters Without BaseContext
Sometimes, you might not want to use BaseContext
. Here’s how to inject simple parameters directly.
Agent Configuration
Configure the agents for the interaction.
config_list
defines the LLM configurations, including the model and API key.UserProxyAgent
simulates user inputs without requiring actual human interaction (set toNEVER
).AssistantAgent
represents the AI agent, configured with the LLM settings.
Register the Function with Direct Parameter Injection
Instead of injecting a full context like Account
, you can directly inject individual parameters, such as the username and password, into a function. This allows for more granular control over the data injected into the function, and still ensures that sensitive information is managed securely.
Here’s how you can set it up:
Initiate the Chat
As before, initiate a chat to test the function.
Assigning Different Contexts to Multiple Agents
You can assign different contexts, such as distinct account data, to different agents within the same group chat. This ensures that each assistant works with its own unique set of data—e.g., one assistant can use alice_account
, while another can use bob_account
.
GroupChat Configuration
Let’s configure a GroupChat
with two assistant agents.
Register Functions for Each Assistant
- For
assistant_1
, we inject thealice_account
context usingDepends(alice_account)
, ensuring that it retrieves the balance for Alice’s account. - For
assistant_2
, we inject thebob_account
context usingDepends(bob_account)
, ensuring that it retrieves the balance for Bob’s account.
Initiate the Chat
Finally, initiate the group chat where both assistants respond by using their respective contexts. Each assistant will handle its own task — one will retrieve Alice’s balance, and the other will retrieve Bob’s balance
Conclusion
In this blog post, we explore Dependency Injection (DI) as a secure and effective way to manage sensitive data in workflows involving LLMs. Dependency Injection ensures that sensitive data, such as passwords or personal information, remains protected by injecting necessary details at runtime instead of exposing them directly to the LLM.
The post provides a comprehensive guide on setting up and using DI with agents, illustrating how to securely retrieve account balances without sharing sensitive data. It includes step-by-step instructions on configuring agents, defining contexts, and using the Depends function to inject account details directly into the functions. Various methods are demonstrated, such as injecting contexts, passing simple parameters, and even managing multiple contexts for different agents in group chats.
By following this guide, developers can create secure and flexible workflows that prevent unauthorized access to sensitive data while leveraging LLMs’ full potential.